Felgo 2.18.1 introduces new components for embedding YouTube videos, for creating material cards and Tinder-like swipe cards. It also simplifies connecting to REST services, with the new HttpRequest component. Felgo 2.18.1 also adds several other fixes and improvements.
Important Note for iOS Live Client: The current store version of the Felgo Live Client app is built with Felgo 2.17.1 and does not include the latest features. If you want to use QML live code reloading with the latest Felgo features on iOS, you can build your own live clients with Live Client Module.
Connect to REST Services with JavaScript Promises and Image Processing from QML
You can now use the HttpRequest type as an alternative to the default XmlHttpRequest. It is available as a singleton item for all components that use import Felgo 3.0:
import Felgo 3.0
import QtQuick 2.0
App {
Component.onCompleted: {
HttpRequest
.get("http://httpbin.org/get")
.timeout(5000)
.then(function(res) {
console.log(res.status);
console.log(JSON.stringify(res.header, null, 4));
console.log(JSON.stringify(res.body, null, 4));
})
.catch(function(err) {
console.log(err.message)
console.log(err.response)
});
}
}
Similar to HttpRequest, which matches the DuperAgent Request type, other DuperAgent features are also available in Felgo with the Http prefix:
- HttpNetworkActivityIndicator – Allows to check for pending requests of HttpRequest, using HttpNetworkActivityIndicator::enabled.
- HttpImageUtils – Offers scaling and cropping of image files before uploading it via DuperAgent’s attach function.
The HttpRequest type also supports response caching of your requests out-of-the-box.
The DuperAgent package, which brings the HttpRequest type, also contains an implementation of the Promises/A+ specification and offers an API similar to the Promises API in ES2017. The Promise type works independently of DuperAgents’s http features:
import Felgo 3.0
import QtQuick 2.0
App {
Component.onCompleted: {
var p1 = Promise.resolve(3);
var p2 = 1337;
var p3 = HttpRequest
.get("http://httpbin.org/get")
.then(function(resp) {
return resp.body;
});
var p4 = Promise.all([p1, p2, p3]);
p4.then(function(values) {
console.log(values[0]); // 3
console.log(values[1]); // 1337
console.log(values[2]); // resp.body
});
}
}
Add QML Material Design Cards and Tinder Swipe Gestures
Create material design cards with the new AppCard. You can also use Tinder-like swipe gesture with cards. With additional AppPaper and AppCardSwipeArea components, you can create fully custom card-like UI elements, that can be swiped in a Tinder-like fashion.
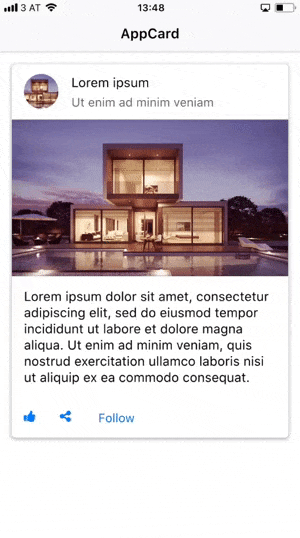
import Felgo 3.0
import QtQuick 2.0
App {
Page {
AppCard {
id: card
width: parent.width
margin: dp(15)
paper.radius: dp(5)
swipeEnabled: true
cardSwipeArea.rotationFactor: 0.05
// If the card is swiped out, this signal is fired with the direction as parameter
cardSwipeArea.onSwipedOut: {
console.debug("card swiped out: " + direction)
}
// … Card content
}
}
}
Embed YouTube Videos on your Qt App
With the YouTubeWebPlayer component, you can now directly embed YouTube videos in your app with a simple QML API.
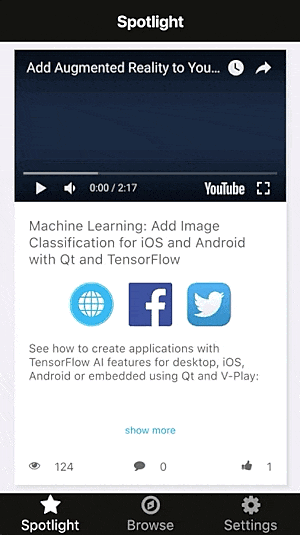
This is how you can use the player in QML:
import Felgo 3.0
App {
NavigationStack {
Page {
title: "YouTube Player"
YouTubeWebPlayer {
videoId: "KQgqTYCfJjM"
autoplay: true
}
}
}
}
The component uses a WebView internally and the YouTube Iframe-Player API. To show how you can use the player in your app, you can have a look at the YouTube Player Demo App. It uses the YouTube Data API to browse playlists and videos of a configured channel.
Have a look at this demo to see how to integrate the Qt WebView module and use the YouTubeWebPlayer to play videos. The demo also shows how to load content from the YouTube Data API via http requests.
QML QSortFilterProxyModel to use Sorting and Filters ListModels
You can now use SortFilterProxyModel, based on QSortFilterProxyModel, to apply filter and sorting settings to your QML ListModel items.
The following example shows the configured entries of the ListModel in a ListPage, and allows to sort the list using the name property:
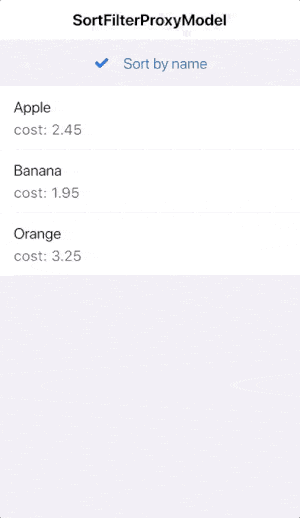
import Felgo 3.0
import QtQuick 2.0
App {
// data model
ListModel {
id: fruitModel
ListElement {
name: "Banana"
cost: 1.95
}
ListElement {
name: "Apple"
cost: 2.45
}
ListElement {
name: "Orange"
cost: 3.25
}
}
// sorted model for list view
SortFilterProxyModel {
id: filteredTodoModel
sourceModel: fruitModel
// configure sorters
sorters: [
StringSorter {
id: nameSorter
roleName: "name"
}]
}
// list page
NavigationStack {
ListPage {
id: listPage
title: "SortFilterProxyModel"
model: filteredTodoModel
delegate: SimpleRow {
text: name
detailText: "cost: "+cost
style.showDisclosure: false
}
// add checkbox to activate sorter as list header
listView.header: AppCheckBox {
text: "Sort by name"
checked: nameSorter.enabled
updateChecked: false
onClicked: nameSorter.enabled = !nameSorter.enabled
anchors.horizontalCenter: parent.horizontalCenter
height: dp(48)
}
} // ListPage
} // NavigationStack
} // App
Combine Multiple Filter and Sorting Settings on QML ListModels
The SortFilterProxyModel helps you to combine multiple filter and sorting settings on a model. You can find a detailed example in our documentation: Advanced SortFilterProxyModel Example.
It also fetches data from a REST API using the new HttpRequest type.
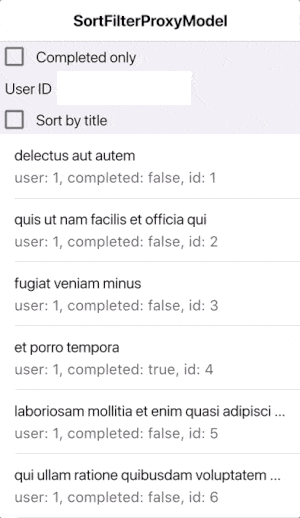
More Features, Improvements and Fixes
Here is a compressed list of further improvements with this update:
- The Page type now features two more signals appeared() and disappeared(). These signals fire when the page becomes active or inactive on a NavigationStack. They are convenience signals to avoid manual checks of Page::isCurrentStackPage.
- Remove focus from SearchBar text field if it gets invisible.
- Fixes a crash in the Felgo Live Client when using WikitudeArView.
- When a device goes online, the App::isOnline property now becomes true only after a short delay. This is required, as otherwise the network adapter might not be ready yet, which can cause immediate network requests to fail.
For a list of additional fixes, please check out the changelog.
More Posts Like This
Machine Learning: Add Image Classification for iOS and Android with Qt and TensorFlow
Qt AR: Why and How to Add Augmented Reality to Your Mobile App
Release 2.18.0: Update to Qt 5.11.1 with QML Compiler and Massive Performance Improvements
Release 2.17.0: Firebase Cloud Storage, Downloadable Resources at Runtime and Native File Access on All Platforms